SDK Ad Integration Advanced Usage
Prerequisites
- Ensure you've already acquired the ExchangeId. If not, please refer to Creating an Exchange Task.
Installing the SDK
Please see SDK Installation for guidance on how to install the SDK.
Native Ads
If you want to display native ads in your app, such as embedding ads into tasks to minimize disruption to the user experience, here's how you can do it:
1. Retrieving Ads
First, you need to load ads. Use the GetMultiTonExchangeAd
function from the SDK to retrieve ads on the frontend.
import { GetMultiTonExchangeAd } from 'ton-ai-sdk'
const exchangeId = 'your_exchange_id'
const limit = 5
GetMultiTonExchangeAd(exchangeId, limit)
.then((ads) => {
if (ads && ads.length > 0) {
// Ads loaded successfully, ready to display at the right time
} else {
// No available ads
}
})
.catch((error) => {
console.error('Failed to load ads:', error)
})
Tips
We recommend retrieving multiple ads at once to improve click-through rates by offering variety, as users may not be interested in a single ad.
The ads object structure is as follows:
{
"ads": [
{
"inExchangeCampaignId": "string", // Target ad ID
"outExchangeCampaignId": "string", // Your current ad ID
"adFormat": "string", //"image" | "video",
"icon": "string", // Advertiser's icon
"text": "string", // Advertising copy
"image": "string", // Ad banner image
"popupImage": "string", // Ad pop-up image
"brandName": "string", // Advertiser's name
"buttonText": "string", // Button text
"url": "string", // Ad redirect link
"destination": {
// Configuration for the ad's redirection
"actionType": "string",
"url": "string"
}
}
]
}
2. Displaying Ads
You can then render parameters from the Ad, like:
- icon
- text
- destination.url
as tasks on the Earn page, for example:
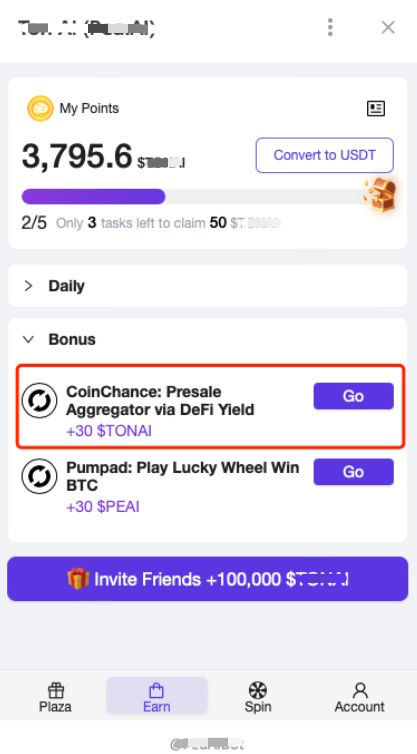
When users click on a task and are redirected, you need to report the Click event using the SDK.
import { SendTonExchangeClickEvent } from 'ton-ai-sdk'
// ad is one object from the ads array
SendTonExchangeClickEvent(ad)
Tips
It's advisable not to immediately mark the task as completed after a user click. Wait a couple of seconds before marking it as complete and reporting the Click event.
Tips
After reporting a Click event, the user will no longer see that ad.