SDK Integration for Native Ads
Prerequisites
- Know where to display ads in your app. If not, please check Ad Integration Examples.
- Have already acquired a BlockId. If not, please look at Get BlockId.
- Refer to SDK Installation to understand how to install the SDK.
Native Ads
We recommend displaying native ads in your app, such as wrapping ads as tasks, which are less intrusive to the user experience.
1. Get Ads
First, load the ads by calling the SDK's GetMultiTonAd
function on the frontend.
import { GetMultiTonAd } from 'ton-ai-sdk'
const blockId = 'your_block_id'
const limit = 5
GetMultiTonAd(blockId, limit)
.then((res) => {
if (res.ads && res.ads.length > 0) {
// Ads loaded successfully, ready to be displayed at the right moment
} else {
// No ads available
}
})
.catch((error) => {
console.error('Failed to load ads:', error)
})
Tips
We suggest getting multiple ads at once to boost click-through rates by offering choices to users who might not be interested in a single ad. During testing, if no ads are retrieved, make sure to set debug: true
when initializing the SDK.
The ads
object structure is as follows:
{
"ads": [
{
"adId": "string", // Ad ID
"adBlockId": "string", // Ad Block ID
"adFormat": "string", //"image" | "video",
"icon": "string", // Advertiser's icon
"text": "string", // Ad copy
"buttonText": "string", // Button text
"url": "string", // Ad redirect link
"destination": {
// Options for ad redirection configuration
"actionType": "string",
"url": "string"
}
}
]
}
2. Wrap Ads
You can render ad parameters like
- icon
- text
as tasks on the Earn page, for example:
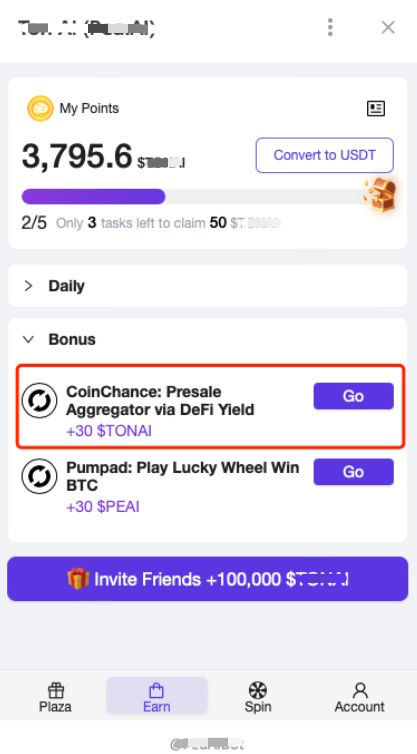
3. Display Ads
When a user clicks on a task, call the SDK to display the ad by passing the clicked ad object ad
.
TonAdPopupShow({
tonAd: ad, // The ad object clicked by the user
countdown: 15, // Optional, default is 10 seconds. Min 10 seconds, max 30 seconds.
autoClose: true, // Default is true, auto-closes the ad after countdown
onAdComplete: (ad) => {
// User has watched the ad, reward can be given here
// sendReward(ad)
},
onAdClick: (ad) => {
// User clicked the ad, reward can be given here
// sendReward(ad)
},
onAdError: (error) => {
// Error encountered during ad playback
console.error(error)
},
onAdClose: (ad) => {
// User manually closed the ad
}
})
Then, based on your product design, choose the right moment to send rewards.
onAdComplete
: Corresponds to CPM mode, meaning users get rewarded after watching the ad.onAdClick
: Corresponds to CPC mode, encouraging user interactions by rewarding clicks.
Tips
If you wish to handle rewards in the backend, refer to the reward-webhook
section in getBlockId.
The ad
object returned from various events is structured as:
{
"adId": "string", // Ad ID
"adBlockId": "string", // Ad Block ID
"adFormat": "string", //"image" | "video",
"icon": "string", // Advertiser's icon
"text": "string", // Ad copy
"buttonText": "string", // Button text
"url": "string", // Ad redirect link
}
Ad Display Effect
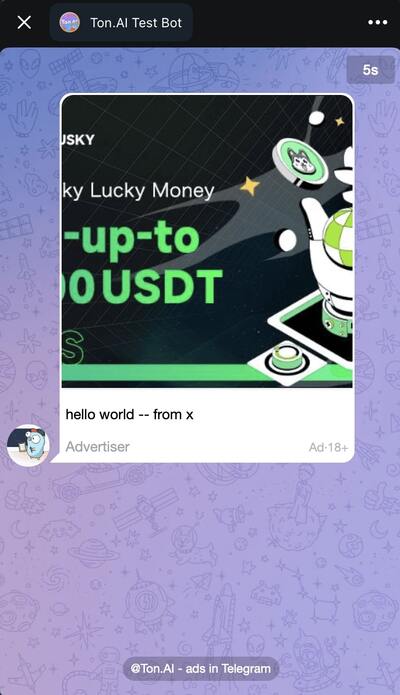